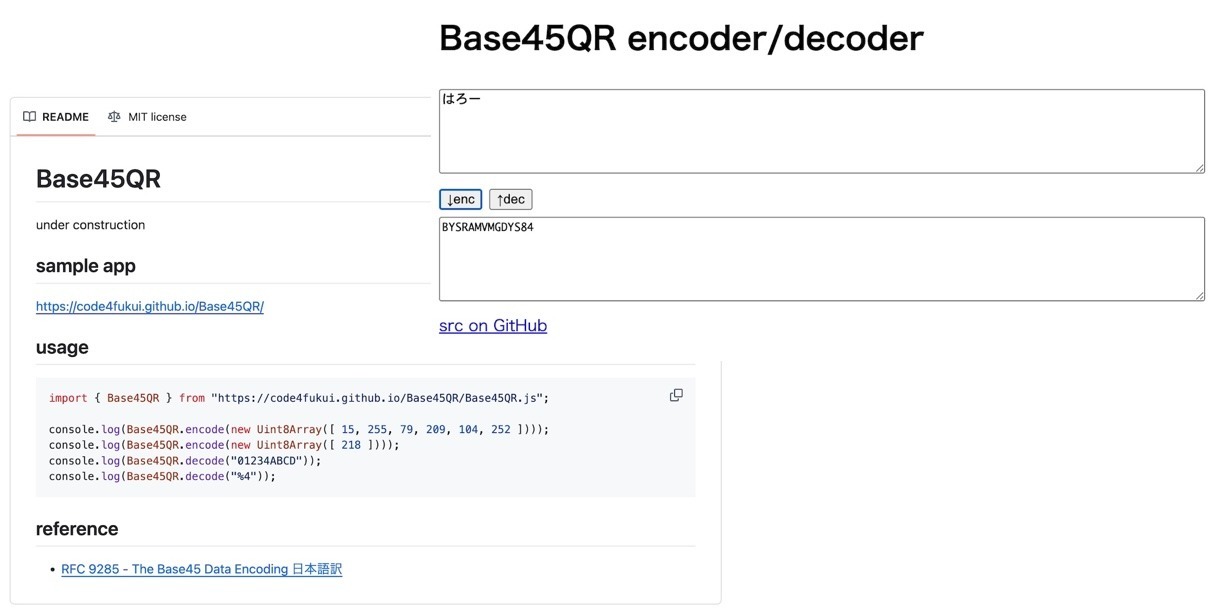
QRコードの数値モードを使った
Base10を作ったついでに、気になったアルファベット大文字とスペースや記号を加えた45種類のアルファニューメリックモード向けのエンコーディング、
Base45QRを実装しました。
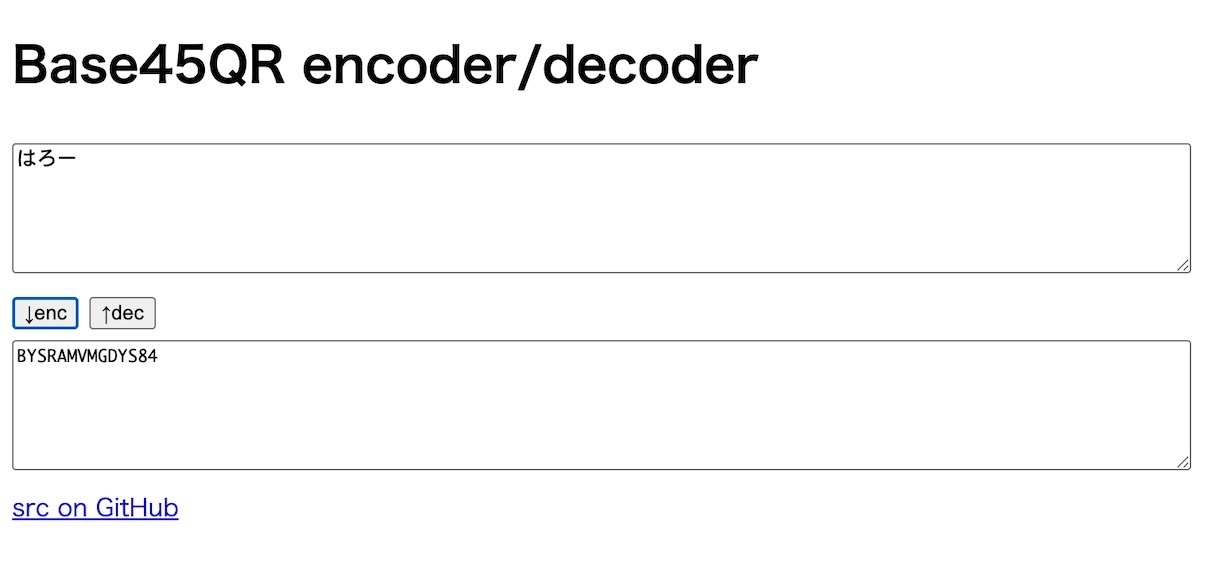
「Base45QR encoder/decoder」 src on GitHub
いつものテストアプリをBase45QR用に作成。上に日本語などを書くと、45種類の文字を使った文字列ができあがります。
公開鍵や、電子署名は、こんな感じの長さになります。
Deno.test("pubkey", () => {
//const prikey = sec.prikey();
//console.log(Base45QR.encode(prikey));
const prikey = Base45QR.decode("7AKYNMVZK2ZLLBB-HT+JDI%JF%JG53%%FLLN3V34 81/D0+G");
const pubkey = sec.pubkey(prikey);
//console.log(Base45QR.encode(pubkey));
t.assertEquals(Base45QR.encode(pubkey), "O63BW84HS23E9Q3H4252I1LC*-LI79RN6N U8U7V.7LSI93G");
const data = new Uint8Array([0]);
const sign = sec.sign(prikey, data);
t.assertEquals(Base45QR.encode(sign), "JUPYZO$+E7M7I62LMS0 QUSG5$HDF4J1S+-52WD4LT :BDQE61Q8+MLP0QVD+LTTH3S:BW%HHB3YG5+:9XCNV-K7XHE.K4WT");
t.assert(sec.verify(sign, pubkey, data));
});
先頭に0が続くと、桁数が変わって長さが変わるBase10と違って、長さは必ず固定なので、複数のデータを組み合わせたい時に便利かも。
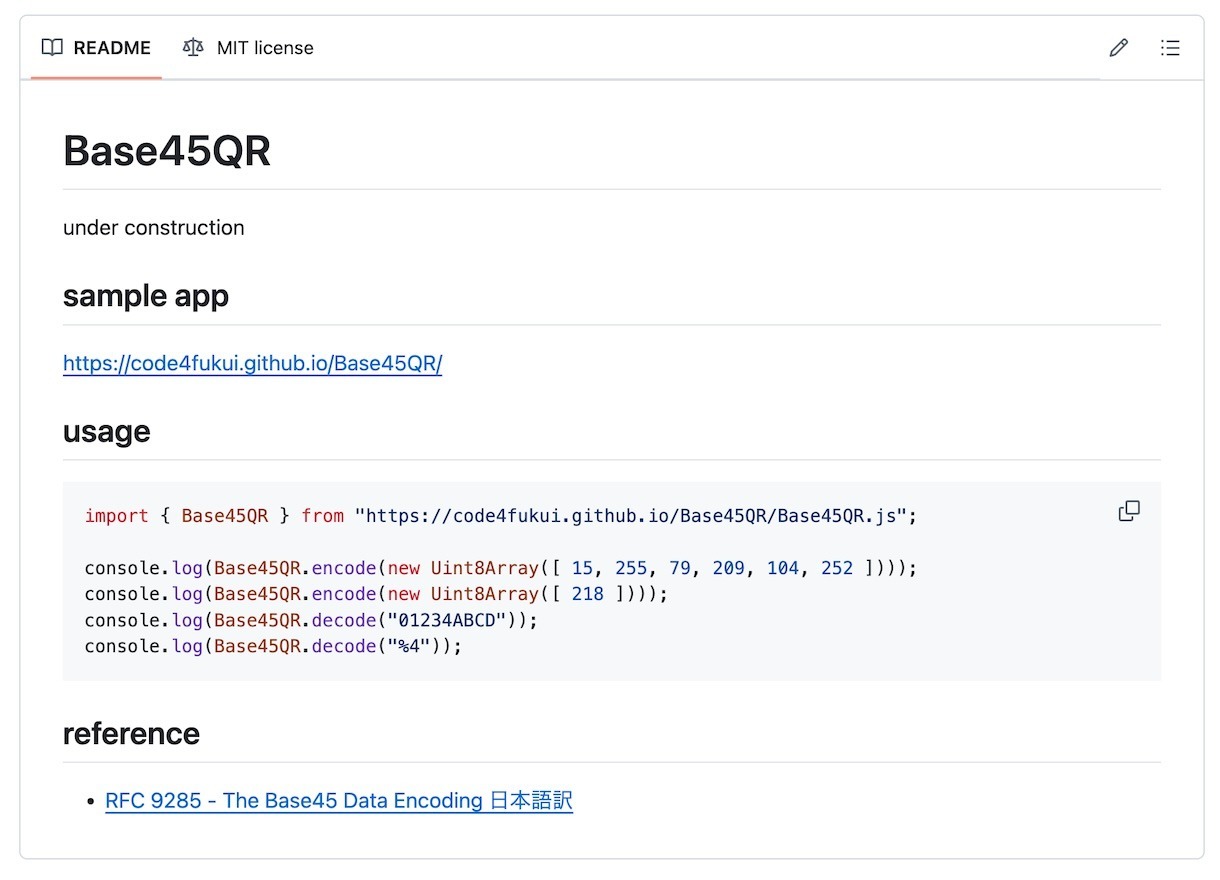
「code4fukui/Base45QR」
メインのコード「Base45QR.js」は、シンプル!
export const BASE45QRS = "0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ $%*+-./:";
const encode = (bin) => {
const txt = [];
for (let i = 0; i < bin.length; i += 2) {
if (i < bin.length - 1) {
let n = (bin[i] << 8) | bin[i + 1];
const n3 = n % 45;
n = (n - n3) / 45;
const n2 = n % 45;
const n1 = (n - n2) / 45;
txt.push(BASE45QRS.charAt(n3));
txt.push(BASE45QRS.charAt(n2));
txt.push(BASE45QRS.charAt(n1));
} else {
const n = bin[i];
const n3 = n % 45;
const n2 = (n - n3) / 45;
txt.push(BASE45QRS.charAt(n3));
txt.push(BASE45QRS.charAt(n2));
}
}
return txt.join("");
};
const decode = (s) => {
const get = (c) => {
const n = BASE45QRS.indexOf(c);
if (n < 0) throw new Error("illegal char");
return n;
};
const bin = [];
for (let i = 0; i < s.length; i += 3) {
if (i < s.length - 2) {
const n3 = get(s[i]);
const n2 = get(s[i + 1]);
const n1 = get(s[i + 2]);
const n = n3 + n2 * 45 + n1 * (45 * 45);
bin.push(n >> 8);
bin.push(n & 0xff);
} else {
const n3 = get(s[i]);
const n2 = get(s[i + 1]);
const n = n3 + n2 * 45;
bin.push(n);
}
}
return new Uint8Array(bin);
}
export const Base45QR = { encode, decode };
QRコード、いろいろと活用しましょう!
links
- 神山まるごと高専生企画のステキイベント「映画と森Night」の空間写真とQRコード向けエンコーディングBase10